Python Basics I
28 Sep 2018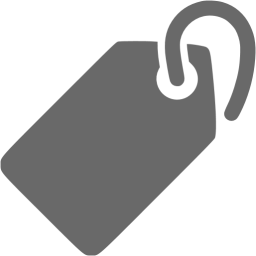
Chapter 1 - Python Basics I
Python is an intuitive language
Python is very intuitive, compared to other programming languages. This is why people say that it is easy to learn for beginners.
Let’s look at the example below
x = 3
if x ==3: print("correct")
correct
Just by reading this code, most people can catch what this code is saying.
The first line says that “x” equals to 3 The second line says that if “x” equals to 3, print the word “correct”
Pretty easy eh?
Code Comments
Code comment is used for explanation from developers. They are ignored during code execution. Code comments can be made after a hashtag. For more multiple code comments, use of three quotations are used. Below is an example
# This is a comment
# The comment section is ignored during the code execution
The multiline comments do not perform anythin,
"""
This is a multi-line comment. It is a special type of string called, Doc-string.
Normally, they are used for detailed explanation of codes, or long comments
"""
'\nThis is a multi-line comment. It is a special type of string called, Doc-string.\nNormally, they are used for detailed explanation of codes, or long comments\n'
Data Types
When you learn English, alphabet is the fundamental unit of knowledge. Python is also a programming “language”. Importance of data types are equivalent to knowing the alphabets.
These are main data types of python:
- Numbers
- Strings
- Boolean
- Lists
- Tuples
- Dictionary
- Set
Let’s cover these data types one by one
Numbers
Numbers include integers, floating numbers and complex numbers. If you have done your mathematics, these terms should be familiar. Also, there are octal and hexadecimal numbers which are not frequently used in general python programming.
- integers –> int
- floating number –> float
- complex number –> complex
Let’s look at them one by one
Integer
# Integer
## Integer is a real number.
#It is a round number with no values after decimal point
x = 50
print(x) # Simple line that orders to show (print) x
type(x) # This shows what the data type of x is
50
int
Variables are assigned by using a single equation sign “=”. They are case sensitive, but using similar variables are not recommended in a complex coding, due to confusion and readability
one = 3
One = 6
print(one, One)
3 6
Float
# Floating Point
# Floating point is a name for a real number.
# It may have numbers after the decimal point
y = 1.4
z = 4.0
print(y)
print(z)
# you can use int() function to make float to integer
print(int(y))
print(int(z))
#You can use float() function to force a number to be considered as a float
my_int = 3
print(type(my_int))
my_int2 = float(3)
print(type(my_int2))
1.4
4.0
1
4
<class 'int'>
<class 'float'>
Complex
# Complex
# Complex is a complex number.
# It is a number that can be expressed in the form of a + bi
# a, b are real numbers while i is the solution of x^2= -1
x = complex(2,4)
y = complex(1,5)
print(x,y, x + y)
(2+4j) (1+5j) (3+9j)
Basic Math
Python has a number of built-in math functions. These can be extended even further by importing the math package or by including any number of other calculation-based packages.
All of the basic arithmetic operations are supported: +, -, /, and . You can create exponents by using * and modular arithmetic is introduced with the mod operator, %.
print ('Addition: ', 2 + 2)
print ('Subtraction: ', 7 - 4)
print ('Multiplication: ', 2 * 5)
print ('Division: ', 10 / 2)
print ('Exponentiation: ', 3**2)
print ('Modulo: ', 15 % 4) # remainder
Addition: 4
Subtraction: 3
Multiplication: 10
Division: 5.0
Exponentiation: 9
Modulo: 3
This also works on variables
first_integer = 4
second_integer = 5
print (first_integer * second_integer)
x = 3
x - 2
20
1
first_integer = 11
second_integer = 3
print (first_integer / second_integer)
3.6666666666666665
Python’s built-in math functions
- abs()
- round()
- max()
- min()
- sum()
These functions all act as you would expect, given their names. Calling abs() on a number will return its absolute value. The round() function will round a number to a specified number of the decimal points (the default is 00 ). Calling max() or min() on a collection of numbers will return, respectively, the maximum or minimum value in the collection. Calling sum() on a collection of numbers will add them all up. If you’re not familiar with how collections of values in Python work, don’t worry! We will cover collections in-depth in the next section.
Strings
string is a data type that lets you include text as a variable. They are defined using single quotes or double quotes
Both are allowed so that we can include apostrophes or quotation marks in a string if we so choose.
You may use \ to include apostrophes or quotation marks into the string
say_hello = "Hello. My name is python"
print(say_hello)
say_bye = 'Bye. It was nice to meet you'
print(say_bye)
my_string = '"Jabberwocky", by Lewis Carroll'
print (my_string)
my_string = "'Twas brillig, and the slithy toves / Did gyre and gimble in the wabe;"
print (my_string)
alt_string = 'He said, \'this is ridiculous.\''
print(alt_string)
Hello. My name is python
Bye. It was nice to meet you
"Jabberwocky", by Lewis Carroll
'Twas brillig, and the slithy toves / Did gyre and gimble in the wabe;
He said, 'this is ridiculous.'
Calculation in Strings
# Addition
first_name = "Harry"
last_name = "Potter"
first_name + last_name
'HarryPotter'
# Multiplication
twice = "likey"
twice*2
'likeylikey'
# Usage
print("="*40)
print("Oh Yeah")
print("="*40)
========================================
Oh Yeah
========================================
Indexing and Slicing
sentence = "wingardium leviosa"
# Python starts from 0
print(sentence[4])
print(sentence[0])
print(sentence[-2])
a
w
s
# Usage
print( sentence[11] + sentence[15] + sentence[13] + sentence[12])
# sentence[0:4] --> 0<= sentence < 4
print( sentence[0:4])
love
wing
# Changing string by indexing
a = "League of Leg nd"
print(a)
# You cannot change string or tuple. These are called imutable data types
a[-3] = "e"
print(a)
League of Leg nd
---------------------------------------------------------------------------
TypeError Traceback (most recent call last)
<ipython-input-56-e0f9681d392f> in <module>()
4
5 # You cannot change string or tuple. These are called imutable data types
----> 6 a[-3] = "e"
7 print(a)
TypeError: 'str' object does not support item assignment
correct = a[:7] + a[7:-3] + "e" + a[-2:]
print(correct)
League of Legend
Formatting Strings
Using the format() method we can add in variable values and generally format our strings.
my_string = "{0} {1}".format('Marco', 'Polo')
print( my_string)
my_string = "{1} {0}".format('Marco', 'Polo')
print (my_string)
Marco Polo
Polo Marco
We use braces ({}) to indicate parts of the string that will be filled in later and we use the arguments of the format() function to provide the values to substitute. The numbers within the braces indicate the index of the value in the format() arguments
print ('insert %s here' % 'value')
insert value here
The % symbol basically cues Python to create a placeholder. Whatever character follows the % (in the string) indicates what sort of type the value put into the placeholder will have. This character is called a conversion type. Once the string has been closed, we need another % that will be followed by the values to insert. In the case of one value, you can just put it there. If you are inserting more than one value, they must be enclosed in a tuple.
print ('There are %s cats in my %s' % (13, 'apartment'))
There are 13 cats in my apartment
Functions related to strings
- count()
- find()
- index()
- join()
- upper() / lower()
- lstrip() / rstrip()
- replace()
- split()
# Returns the number of character in the string
a = "Python is fun"
a.count("n")
2
# Returns the value of first position. If does not exist, return -1
print(a.find("n"))
print(a.find("k"))
5
-1
# Returns the index number
print(a.index("s"))
8
# Insert string a between 1 and 2
print(a.join("12"))
1Python is fun2
# Change the characters to uppercase or lowercase
print(a.upper(), a.lower())
PYTHON IS FUN python is fun
# Deleting spaces in the left of right side of the string
b = " I have lots of space "
print(b.lstrip())
print(b.rstrip())
# Deleting spaces in both side of string
print(b.strip())
I have lots of space
I have lots of space
I have lots of space
# Replace
a.replace("fun","horrible")
'Python is horrible'
# Slicing
print(a.split())
# Another example
k = "I/do/not/like/your/shirt"
print(k.split("/"))
print(k.split("t"))
['Python', 'is', 'fun']
['I', 'do', 'not', 'like', 'your', 'shirt']
['I/do/no', '/like/your/shir', '']
Encoding
Normal strings in python are stored internally as 8-bit (= 1byte) ASCII. On the other hand, unicode uses 16-bit system (= 2byte) and it can store a more varied set of characters.
Chinese characters and Korean characters cannot be represented in ASCII code.
UTF-8 strings are stored as 8 bit (= 1byte) just like ASCII, but it works as an extension of ASCII. It can express Korean also.
Frequent errors that you can encounter
a = "안녕"
b = a.encode("utf-8")
print(b)
c = b.decode("utf-8")
print(a)
b'\xec\x95\x88\xeb\x85\x95'
안녕
str – encode () –> bytes – decode() –> str
Boolean
Boolean is related to logical operators, and takes one of two values only: True or False (True = 1, False = 0)
Default Boolean values follows:
Values | True of False |
---|---|
“python” | True |
”” | False |
[] | False |
{} | False |
() | False |
0 | False |
1 | True |
[1,2,3] | True |
None | False |
Mostly, empty list,tuple,dictionaries are False. If not, they are True. In terms of numbers, 0 is False while 1 is true.
print(0 == 5)
False
and (&) , or ( | ), not
# And
print(True & True) # True and True
print(True & False) # True and False
print(False & False) # False and False
print("="*30)
# Or
print(True | True) # True or True
print(True | False) # True or False
print(False | False) # False or False
print("="*30)
# Not
print(not True)
print(not False)
True
False
False
==============================
True
True
False
==============================
False
True
Lists
List is an ordered collection of objects that can contain any data type. List can be defined using brackets [ ]
eiffel_tower = ["Paris","France","1889",300.65, 324]
We can access and index the list by using brackets as well. In order to select an individual element, simply type the list name followed by the index of the item you are looking for in braces.
eiffel_tower[3]
300.65
Indexing in Python starts from 00 . If you have a list of length nn , the first element of the list is at index 00 , the second element is at index 11 , and so on and so forth. The final element of the list will be at index n−1n−1 . Be careful! Trying to access a non-existent index will cause an error.
We can see the number of elements in a list by calling the len() function
print(len(eiffel_tower))
5
we can update and change list by accessing an index. List is mutable unlike strings meaning you can change them. However, once a string or other immutable data type has been created, it cannot be directly modified without creating an entirely new object.
print(eiffel_tower[4])
eiffel_tower[4] = 320
print(eiffel_tower[4])
324
320
If you want to put two lists together, they can be combined with a + symbol.
# You can put list in a list!
list1 = ["i", 3, 4.30, [1,2,"harry"], "you"]
list2 = ["hello",4.5, 3.0]
list3 = list1 + list2
print(list3)
['i', 3, 4.3, [1, 2, 'harry'], 'you', 'hello', 4.5, 3.0]
Indexing and Slicing
Lists have similar mechanism of indexing and slicing just as strings do
starwars = ["may","the","Force","be","with","you"]
print(starwars[2:4])
print(starwars[1:])
print(starwars[:3])
['Force', 'be']
['the', 'Force', 'be', 'with', 'you']
['may', 'the', 'Force']
['may', 'the', 'Force', 'be', 'with', 'you']
You can also add a third component to slicing. Instead of simply indicating the first and final parts of your slice, you can specify the step size that you want to take. So instead of taking every single element, you can take every other element.
confusing = ["may",1,"the",2,"Force",3,"be",4,"with",5,"you"]
print(confusing[0:10:2])
print(confusing[::2])
print(confusing[::-1])
print(confusing[::-2])
['may', 'the', 'Force', 'be', 'with']
['may', 'the', 'Force', 'be', 'with', 'you']
['you', 5, 'with', 4, 'be', 3, 'Force', 2, 'the', 1, 'may']
['you', 'with', 'be', 'Force', 'the', 'may']
Functions related with lists
- append
- sort
- reverse
- index
- insert
- remove
- pop
- count
- extend
# append
a = [1,2,3]
a.append(4)
a
[1, 2, 3, 4]
# sort
a = [2,5,3,4,7,1,3]
a.sort()
print(a)
b = ["c","d","b","w","f","r"]
b.sort()
print(b)
[1, 2, 3, 3, 4, 5, 7]
['b', 'c', 'd', 'f', 'r', 'w']
c = [6,4,8,"e",3,"b","c"]
# This does not work
c.sort()
---------------------------------------------------------------------------
TypeError Traceback (most recent call last)
<ipython-input-122-ff5aa901bedc> in <module>()
1 c = [6,4,8,"e",3,"b","c"]
2 # This does not work
----> 3 c.sort()
TypeError: unorderable types: str() < int()
a = ["a","c","b"]
a.reverse()
a
['b', 'c', 'a']
a = [1,5,3,7]
a.insert(3,6)
a
[1, 5, 3, 6, 7]
a = [1,2,3,4,5,6,7]
a.remove(5)
a
[1, 2, 3, 4, 6, 7]
a = [1,4,6]
a.pop()
a
[1, 4]
a = [1,4,7,3,5,6,"d"]
a.count("d")
1
a = [1,2,3,4,5]
a.extend(["d","5","d",5])
a
[1, 2, 3, 4, 5, 'd', '5', 'd', 5]
Tuple
Tuple is similar to list. The difference between tuple and list is that tuple is immutable. The data cannot be changed once it has been generated.
t1 = ()
# When tuple only has one value, comma must be follwed
t2 = (1,)
t3 = (1)
print(type(t2), type(t3))
# When assigning variable with tuple, brackets can be skipped
t4 = (1,2,3)
t5 = 1,2,3
print(type(t4), type(t5))
<class 'tuple'> <class 'int'>
<class 'tuple'> <class 'tuple'>
Tuple indexing and slicing is similar to list
tup = (1,4,7,4,"d","d2",2,"4")
print(tup[4])
print(tup[2:])
d
(7, 4, 'd', 'd2', 2, '4')
Tuple addition and multiplication
tuple1 = (1,2,5)
tuple2 = (4,6,7)
tuple3 = tuple1 + tuple2
print(tuple3)
print(tuple2*4)
(1, 2, 5, 4, 6, 7)
(4, 6, 7, 4, 6, 7, 4, 6, 7, 4, 6, 7)
Dictionary
Dictionary is an important data structure in python. Dictionaries are defined with a combination of curly braces {} and colons : The braces define the beginning and end of a dictionary and the colons indicate key-value pairs. A dictionary is essentially a set of key-value pairs. The key of any entry must be an immutable data type. This makes both strings and tuples candidates. Keys can be both added and deleted.
In the following example, we have a dictionary composed of key-value pairs where the key is a genre of fiction (string) and the value is a list of books (list) within that genre. Since a collection is still considered a single entity, we can use one to collect multiple variables or values into one key-value pair.
Dictionary Example
Key | Value |
---|---|
name | Jake |
phone | 01055999411 |
birth | 19941125 |
Personal_Info = {"name":"Jake",
"phone":"01055999411",
"birth":"19941125"}
Dictionary Usage & Manipulation
Idols = {"Twice": ["Jihyo","Nayeon","Jungyeon","Momo","Sana","Mina","Dahyun","Chaeyoung","Tzuyu"],
"Blackpink": ["Jisu","Jennie","Rose","Lisa"],
"Bigbang": ["GD","TOP","Taeyang","Daesung","Seungri"],
"BTS": ["RM","Suga","Jin","J-Hope","Jimin","V","Jungkook"]}
After defining a dictionary, we can access any values by indicating its key in brackets
# Using Key to retrieve values
print(Idols["BTS"])
print(Idols["Twice"])
['RM', 'Suga', 'Jin', 'J-Hope', 'Jimin', 'V', 'Jungkook']
['Jihyo', 'Nayeon', 'Jungyeon', 'Momo', 'Sana', 'Mina', 'Dahyun', 'Chaeyoung', 'Tzuyu']
Dictionary can be changed by the value associated with a given key
Idols["Bigbang"] = ["GD","Top","Daesung","Taeyang"]
print(Idols["Bigbang"])
['GD', 'Top', 'Daesung', 'Taeyang']
Cautions when making dictionary
Dictionary Key is a unique value. This means that same key cannot be used again. Also, list cannot be used in the key. This will cause error
# key cannot be used again (there are two "5"s in the key)
xdict = {5:"a", 5:"b"}
xdict # only returns 5:"b"
{5: 'b'}
Dictionary related functions
- keys
- values
- items
- clear
- get
- in
# Retrieve only "keys" from the dictionary
Idols.keys()
dict_keys(['BTS', 'Twice', 'Bigbang', 'Blackpink'])
# Retrieve only "values" from the dictionary
Idols.values()
dict_values([['RM', 'Suga', 'Jin', 'J-Hope', 'Jimin', 'V', 'Jungkook'], ['Jihyo', 'Nayeon', 'Jungyeon', 'Momo', 'Sana', 'Mina', 'Dahyun', 'Chaeyoung', 'Tzuyu'], ['GD', 'Top', 'Daesung', 'Taeyang'], ['Jisu', 'Jennie', 'Rose', 'Lisa']])
# Retrieve "keys" and "values" pairs from the dictionary
Idols.items()
dict_items([('BTS', ['RM', 'Suga', 'Jin', 'J-Hope', 'Jimin', 'V', 'Jungkook']), ('Twice', ['Jihyo', 'Nayeon', 'Jungyeon', 'Momo', 'Sana', 'Mina', 'Dahyun', 'Chaeyoung', 'Tzuyu']), ('Bigbang', ['GD', 'Top', 'Daesung', 'Taeyang']), ('Blackpink', ['Jisu', 'Jennie', 'Rose', 'Lisa'])])
# Delete everything in the dictionary
Personal_Info.clear()
Personal_Info
{}
# Getting values with key
Idols.get("Blackpink")
['Jisu', 'Jennie', 'Rose', 'Lisa']
# Checking if key is in dictionary
"BtoB" in Idols
False
"Bigbang" in Idols
True
Set
Set is made with set() function. Set does not allow repetitive values, and it is unordered.
s1 = set([1,2,3])
s2 = set("This is set")
print(s1, s2)
{1, 2, 3} {'i', 'h', 'T', 't', 's', ' ', 'e'}
Because set does not have orders, it cannot be indexed nor sliced. In order to perform this, they have to be converted to tuples or lists.
l2 = list(s2)
print(l2)
print(l2[4])
['i', 'h', 'T', 't', 's', ' ', 'e']
s
Set is useful when calculating intersection and union
a = set([1,2,5,7,8,9])
b = set([2,6,3,8,4])
# Intersection
print(a & b)
print(a.intersection(b))
# union
print( a | b)
print(a.union(b))
# subtraction
print( a - b)
print( a.difference(b))
{8, 2}
{8, 2}
{1, 2, 3, 4, 5, 6, 7, 8, 9}
{1, 2, 3, 4, 5, 6, 7, 8, 9}
{1, 5, 9, 7}
{1, 5, 9, 7}
Set related functions
- add
- update
- remove
# Adding a value
s1 = set([1,4,6,7])
s1.add(9)
print(s1)
{1, 4, 9, 6, 7}
# Adding multiple values
s2 = set([5,8,3])
s2.update([1,4,5,6])
print(s2)
{1, 3, 4, 5, 6, 8}
# Removing a value
s3 = set([2,5,8,1])
s3.remove(2)
print(s3)
{8, 1, 5}